- Overview
fio.js
- Library Integrationenroll()
- Enroll New Userauthenticate()
- Recognize UserrestartSession()
- Request New User Session- Error Codes
- HTML Integration Boilerplate
- Community Contributed Tutorials
Overview
The FACEIO Widget is a simple and elegant interface to provide secure facial authentication experience to your users via simple calls to the enroll()
& authenticate()
methods. The Widget is powered by the fio.js
JavaScript library, which is simple enough to integrate in a matter of minutes while being flexible enough to support highly customized setups. Once implemented on your website or web-based application, you'll be able to authenticate your existing users, enroll new ones securely, with maximum convenience on their favorite browser, and at real-time thanks to passwordless experience powered by face recognition.
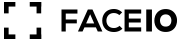
TIP Implementing FACEIO for the first time? Try our 5 Minutes Setup Guide.
fio.js
Library Integration
fio.js
works with regular webcams, and smartphones frontal camera on all modern browsers, does not require biometric sensors, and works seemingly with all websites and web applications regardless of the underlying front-end technology used (ie. React, Vue, jQuery, Vanilla Javascript, static HTML, etc.) or server-side language or framework (eg. PHP, Python, Node.js, Rust, Elixir, etc.).
It’s super quick to get FACEIO Up & Running with just few lines of code. Follow the walkthrough below to implement fio.js
on your site.
Step 1 - Import fio.js
to your site
Paste the snippet below before the closing </body>
tag of the HTML page(s) you want to instantiate fio.js
from...
fio.js
import snippet. The fio.js
library can be imported by linking to faceio’s CDN resource.
<div id="faceio-modal"></div> <script src="https://cdn.faceio.net/fio.js"></script>
The snippet above loads fio.js
asynchronously, so it won’t affect pages load speed. A typical integration in a lambda HTML page should look as follows:
fio.js
<html> <head> <title>Sign-In via Face Recognition</title> </head> <body> <div id="faceio-modal"></div> <script src="https://cdn.faceio.net/fio.js"></script> </body> </html>
💡 Jump to the Boilerplate Section below for the full, lambda HTML template to copy, modify or test on your server...
👉 Always load fio.js
directly from cdn.faceio.net. You can’t self-host it. This will prevent you from receiving bug fixes and new features.
👉 Prefer NPM? Add fio.js
as an NPM dependency using our Official NPM Package, and add it to your own build workflow.
💡 Take a look to our community contributed tutorials listed below. They should help you implement fio.js
on your website or web application using your favorite JavaScript framework whether it is React, Vue, Angular, Next or Vanilla JavaScript.
Step 2 - Instantiate a New faceio
Object
To Initialize fio.js
, simply instantiate a new faceIO()
object and pass your application Public ID as follows. You can also insert this snippet just below the import code shown in Step 1. Again, refer to the HTML Boilerplate for the full integration code sample.
faceIO()
object
<script type="text/javascript"> /* Instantiate fio.js with your application Public ID */ const faceio = new faceIO("app-public-id"); </script>
👉 Your application Public ID is located at the Application Manager on the FACEIO Console. Before you continue, make sure you've:
Created a new FACEIO application, selected a Facial Recognition Engine and activated your application. This is easily done on the FACEIO Console.
Reviewed the Security Options available on the Application Manager. Options includes PIN code requirements, Domain origin and/or country code restrictions, etc. as documented in our Security Best Practices guide.
Reviewed our Privacy Recommendations such as safeguarding and deleting the Unique Facial ID assigned to each enrolled user on your application as stated in our Applications Best Practices guide.
Step 3 - Invoke the Widget
👏Congratulations. You have fio.js
Up & Running. To start the facial recognition process, simply call enroll()
or authenticate()
, the only two exported methods of the faceIO()
class you instantiated earlier. enroll()
is for on-boarding new users (enrollment) while authenticate()
is for authenticating previously enrolled users (Identification/Sign-in).
Before we continue documenting these methods, a typical HTML page implementing fio.js
for the first time should look as follows:
fio.js
for the first time before invoking the enroll()
or authenticate()
methods:
<html> <head> <title>Sign-In or Enroll via Face Recognition</title> </head> <body> <button onclick="enrollNewUser()">Enroll New User</button> <button onclick="authenticateUser()">Authenticate User</button> <div id="faceio-modal"></div> <script src="https://cdn.faceio.net/fio.js"></script> <script type="text/javascript"> // Instantiate fio.js with your application's Public ID const faceio = new faceIO("app-public-id"); function enrollNewUser(){ // call to faceio.enroll() here will automatically trigger the on-boarding process } function authenticateUser(){ // call to faceio.authenticate() here will automatically trigger the facial authentication process } function handleError(errCode){ // Handle error here } </script> </body> </html>
TIP The HTML Boilerplate below, should serve as a reference when implementing fio.js
on your website for the first time.
enroll()
- Enroll New User
Syntax
promise = faceio.enroll({parameters}) promise .then(userInfo => { /* User successfully enrolled */ }) .catch(errCode => { /* handle the error */ })
Alias
enrol()
, register()
, record()
Description
The
enroll()
method let you transactionally enroll new users via face recognition on your FACEIO Application . A process better known as on-boarding. It is the equivalent implementation of a standard register/sign-up function in a traditional password managed, authentication system. Effective call toenroll()
, will trigger the FACEIO Widget, ask for user’s consent (if not yet granted), request access (if not yet granted) to the browser’s Webcam/Frontal camera stream, and finally extract & index the facial features of the enrolled user for future authentication purposes.Facial vectors are stored as an array of floating point numbers. The data is meaningless on its own, effectively acting as a hash, and cannot be reverse engineered. Only your application with its encryption key have access to the currently built index. You can manage, download, grab your encryption key as well as collect analytics on this index via the Application Manager on the FACEIO Console.
enroll()
is a two step operation. The first step is for collecting user’s consent if not yet granted by your host application, while the second step is for indexing the facial features of the user being enrolled, and requires only two frames to operate making it extremely bandwidth efficient and suitable for use in slow networks. Once the facial features collected, the user is invited to input a PIN code of his choice which must be at least 4 digits long (and no more than 16 digits long). On future authentication of this particular user, he is then required to confirm his PIN code whenever a collision occurs (e.g. two extremely similar faces are reported which might happen on very large indexes, or when the same user has enrolled twice), a face match is completed with a confidence score slightly lower than 99% threshold or when your application always enforce PIN code confirmation in order for future authentication of this particular user to succeed.enroll()
takes a number of Optional Parameters includinglocale
for the interaction language,permissionTimeout
which correspond to the number of seconds to wait for the user to grant camera access, but more importantly,enroll()
accept apayload
which simply put, an arbitrary set of data you can link to this particular user such as an Email Address, Name, ID, etc. This payload will be forwarded back to you upon successful future authentication of this particular user.enroll()
returns a Promise whose fulfillment handler receives auserInfo
object when the user has successfully been enrolled. The most important member of theuserInfo
object, is the Facial ID which is, an Unique Identifier assigned to the enrolled user. This Facial ID could serve as a lookup key on your backend for example to fetch data linked to this particular user on future authentication. Details of theuserInfo
object are documented in the Return Value section below. If the user denies camera access permission, or reject the Terms of Use for example, the promise is then rejected with the corresponding error code such asfioErrCode.PERMISSION_REFUSED
orfioErrCode.TERMS_NOT_ACCEPTED
respectively. Refer to the Error Codes section below for the full list of all possible error codes.Finally, if you managed to register a Webhook handler for your application on the FACEIO Console,
enroll()
will make a POST request to the URL you configured for the Webhook after each successful enrollment. Webhooks allows you to receive data and get notified at real-time about the ongoing events during the interaction of the FACEIO Widget with your users in-order to keep your application backend up-to-date & synchronized. FACEIO uses Webhooks to let your system know when such events happen. Details of these events, POST data, and backend synchronization are discussed in details in the Webhooks documentation.
Parameters
enroll()
takes a single, optional parameters
object with the properties to be configured. The table below lists all possible properties of the parameters
object:
Property Name | Type | Default Value | Description |
---|---|---|---|
payload |
Any Serializable JSON | NULL | An arbitrary set of data, you want to associate with the user being enrolled. Example of useful payloads includes Email Address, Name, ID, Token, and so on. This payload will be forwarded back to you upon successful future authentication of this particular user. Payloads data are JSON encoded before saved out, and transferred; therefore you should Base64 Encode any non ASCII string or Binary data your want to associate with a particular user. Maximum payload size per user is set to 16KB. |
permissionTimeout |
Number | 27 Seconds | Total number of seconds to wait for the user to grant camera access permission. Passing this delay, the ongoing enroll() operation is aborted and the promise is rejected with the fioErrCode.PERMISSION_REFUSED error code. |
termsTimeout |
Number | 10 Minutes | Total number of minutes to wait for the user to accept FACEIO/Host Application Terms of Service. Passing this delay, the ongoing enroll() operation is aborted and the promise is rejected with the fioErrCode.TERMS_NOT_ACCEPTED error code. |
idleTimeout |
Number | 27 Seconds | Total number of seconds to wait before giving up if no faces were detected during the enrollment process. Passing this delay, the ongoing operation is aborted and the promise is rejected with the fioErrCode.NO_FACES_DETECTED error code. |
replyTimeout |
Number | 40 Seconds | Total number of seconds to wait before giving up if the remote FACEIO processing node does not return a response (a very unlikely scenario). Passing this delay, the ongoing operation is aborted and the promise is rejected with the fioErrCode.TIMEOUT error code. |
enrollIntroTimeout |
Number | 12 Seconds | Enrollment Widget introduction/instruction screen display delay. |
locale |
String | auto | Default interaction language for the Widget display. If this value is missing or set to auto, then the interaction language will be deducted from the Accept-Language HTTP request header. Otherwise, just pass the BCP 47, two letter, language code of your choice (en, ar, es, ja, de, etc.). If the requested interaction language is not supported, the fallback, default interaction language is US English: en-us. |
userConsent |
Boolean | false | If you have already been granted user consent before enrollment (eg. When the user create a new account on your Website and accept your terms), you can set this parameter to true. In which case, the Terms of Use consent screen is not showed to the end user being enrolled. It is your responsibility to explicitly ask for consent before enrolling a new user. For additional information, please consult our Privacy Best Practices guides for applications. |
Return Value
A Promise whose fulfillment handler receives a userInfo
object when the user has successfully been enrolled. On failure, the promise is rejected with one of the possible error codes listed below.
The table below lists all fields of the userInfo
object returned to your web application by enroll()
when its promise is fulfilled:
Property Name | Type | Description |
---|---|---|
facialId |
UUID (String) |
An identifier assigned anonymously by the underlying facial recognition engine to this particular user. The Facial ID is discussed in details here. |
timestamp |
Timestamp (String) |
ISO 8601, enrollment completion date & time. |
details |
Object | An object with two fields: gender and age which respectively corresponds to the Gender and Age approximation of the enrolled user. |
Example
Back to our HTML/JS integration sample, implementing enroll()
on your client-side JavaScript should look as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | // Instantiate a new faceIO object with your application's Public ID const faceio = new faceIO("app-public-id"); function enrollNewUser(){ faceio.enroll({ "locale": "auto", // Default user locale "payload": { /* The payload we want to associate with this particular user which is forwarded back to us upon future authentication of this user.*/ "whoami": 123456, // Dummy ID linked to this particular user "email": "[email protected]" } }).then(userInfo => { // User Successfully Enrolled! alert( `User Successfully Enrolled! Details: Unique Facial ID: ${userInfo.facialId} Enrollment Date: ${userInfo.timestamp} Gender: ${userInfo.details.gender} Age Approximation: ${userInfo.details.age}` ); console.log(userInfo); // handle success, save the facial ID (userInfo.facialId), redirect to the dashboard... }).catch(errCode => { // Something went wrong during enrollment, log the failure handleError(errCode); }) } |
enroll()
method from your client-side JavaScript.
Effective call to
enroll()
is made on line 4 of the snippet above. This will trigger the onboarding process, request user consent (if not granted yet via theuserConsent
parameter), ask for camera access permission, and finally start the facial features extraction process which requires only two frames to operate.Payload data is linked to this particular user via the
payload
parameter on line 6. In this snippet, it is an object holding a dummy email address and an ID. This payload will be forwarded back to you upon successful future authentication of this particular user so you can clearly identify this user on your backend for example.On successful enrollment, the promise is resolved (line 13) with a
userInfo
object holding the Unique Facial ID assigned to this particular user, which will be same for each successful future authentication of this user. Included also with theuserInfo
object, enrollmenttimestamps
,gender
andage
approximation of the enrolled user.Error handing is done on line 24. Jump to the error codes section below for the list of all possible error codes.
Finally, the HTML Integration Boilerplate below, should serve as a reference when implementing FACEIO on your website for the first time.
authenticate()
- Identify/Recognize Enrolled Users
Syntax
promise = faceio.authenticate({parameters}) promise .then(userData => { /* User successfully authenticated/identified */ }) .catch(errCode => { /* handle the error */ })
Alias
auth()
, recognize()
identify()
Description
The
authenticate()
method let you securely authenticate (recognize) previously enrolled users on your application. It is the equivalent implementation of a standard login/sign-in function in a traditional password managed, authentication system. Effective call toauthenticate()
, will trigger the FACEIO Widget, request access (if not yet granted) to the browser’s Webcam/Frontal camera stream, and finally start the facial recognition process.authenticate()
requires only a single frame to operate, takes less than 100 milliseconds to execute on the average, and is extremely bandwidth efficient making it suitable for use in slow networks. Depending on your app’s security configuration, once the target user has been successfully identified by the underlying facial recognition engine, he has to confirm his PIN code (freely chosen during enrollment) in order for theauthenticate()
method to succeed and thus Promise to be resolved.authenticate()
takes a number of Optional Parameters includingpermissionTimeout
which correspond to the number of seconds to wait for the user to grant camera access,locale
for the interaction language, etc. all documented below.authenticate()
returns a Promise whose fulfillment handler receives auserData
object when the user has successfully been identified. The most important fields are:payload
, which is the arbitrary data you have already linked (if any) to this particular user during his enrollment via thepayload
parameter theenroll()
method takes, and Facial ID, which is an Unique Identifier assigned to this particular user during his enrollment. This Facial ID alongside thepayload
data could serve as a lookup key on your backend to fetch data linked to this particular user on each successful authentication for example. Details of theuserInfo
object are documented in the Return Value section below. If the user denies camera access permission example, the promise is then rejected with the corresponding error code such asfioErrCode.PERMISSION_REFUSED
orfioErrCode.TERMS_NOT_ACCEPTED
. Refer to the Error Codes section below for the full list of all possible error codes.
Parameters
authenticate()
takes a single, optional parameters
object with the properties to be configured. The table below lists all possible properties of the parameters
object:
Property Name | Type | Default Value | Description |
---|---|---|---|
permissionTimeout |
Number | 27 Seconds | Total number of seconds to wait for the user to grant camera access permission. Passing this delay, the ongoing authenticate() operation is aborted and the promise is rejected with the fioErrCode.PERMISSION_REFUSED error code. |
idleTimeout |
Number | 27 Seconds | Total number of seconds to wait before giving up if no faces were detected during the authentication process. Passing this delay, the ongoing operation is aborted and the promise is rejected with the fioErrCode.NO_FACES_DETECTED error code. |
replyTimeout |
Number | 40 Seconds | Total number of seconds to wait before giving up if the remote FACEIO processing node does not return a response (a very unlikely scenario). Passing this delay, the ongoing operation is aborted and the promise is rejected with the fioErrCode.TIMEOUT error code. |
locale |
String | auto | Default interaction language of the FACEIO Widget. If this value is missing or set to auto, then the interaction language will be deducted from the Accept-Language HTTP request header. Otherwise, just pass the BCP 47, two letter, language code of your choice (en, ar, es, ja, de, etc.). If the requested interaction language is not supported, the fallback, default interaction language is US English: en-us. |
Return Value
A Promise whose fulfillment handler receives a userData
object when the user has successfully been identified. On failure, the promise is rejected with one of the possible error codes listed below.
The table below lists all fields of the userData
object returned to your web application by authenticate()
when its promise is fulfilled:
Property Name | Type | Description |
---|---|---|
payload |
Any | The arbitrary data you have already linked (if any) to this particular user during his enrollment via the payload parameter of the enroll() method. |
facialId |
UUID (String) |
The Unique Identifier assigned to this particular user. FACEIO recommend that your rely on this Facial ID (which is automatically generated for each enrolled user on your application), if you plan to uniquely identify all enrolled users on your backend for example. The Facial ID is discussed in details here. |
Example
Back to our HTML/JS integration sample, implementing authenticate()
should look as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | const faceio = new faceIO("app-public-id"); // Initialize with your application's Public ID function authenticateUser(){ faceio.authenticate({ "locale": "auto" // Default user locale }).then(userData => { console.log("Success, user identified") // Grab the facial ID linked to this particular user which will be same // for each of his successful future authentication. FACEIO recommend // that your rely on this Facial ID if you plan to uniquely identify // all enrolled users on your backend for example. console.log("Linked facial Id: " + userData.facialId) // Grab the arbitrary data you have already linked (if any) to this particular user // during his enrollment via the payload parameter of the enroll() method. console.log("Payload: " + JSON.stringify(userData.payload)) // {"whoami": 123456, "email": "[email protected]"} from the enroll() example above }).catch(errCode => { handleError(errCode) }) } |
authenticate()
method from your client-side JavaScript.
Effective call to
authenticate()
is made on line 3 of the snippet above. This will trigger the authentication process after requesting camera access permission (if not yet granted) by the end user.On successful authentication, when the user’s face has been clearly identified, the promise is resolved with a
userData
object holding the Unique Facial ID assigned to this particular user during his enrollment, alongside with thepayload
data you have already linked to this particular user if any during his enrollment.Error handing is done on line 16. Jump to the error codes section below for the list of all possible error codes.
Finally, the HTML Integration Boilerplate below, should serve as a reference when implementing FACEIO on your website for the first time.
restartSession()
- Request New User Session
Syntax
boolean = faceio.restartSession({})
Description
Purge the current session and request a new one. By default, sessions in FACEIO starts following the first call to the
enroll()
orauthenticate()
method whichever comes first, and are immutable. After successful call to this method, the old session is discarded, a new one is created, and you can perform another round of calls toenroll()
orauthenticate()
for the same user without reloading (refreshing) the entire HTML page or relying on an external JavaScript function such aslocation.reload()
to do so. Dynamic session generation via this method are available to the Premium Plans only. Think of upgrading your Freemium application from the FACEIO Console first before calling this method on your frontend.
Parameters
NoneReturn Value
true
if the request for a new session have been granted, and ready for another round of calls to enroll()
or authenticate()
for the same user. false
otherwise.
Error Codes
The table below lists all possible error codes that are returned from either the enroll()
or the authenticate()
methods when their promises are rejected respectively.
Error Code | Description | Effect on enroll() or authenticate() |
---|---|---|
fioErrCode.PERMISSION_REFUSED |
Access to the camera stream was denied by the end user. | Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.TERMS_NOT_ACCEPTED |
Terms & Conditions set out by FACEIO/host application rejected by the end user. | Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.SESSION_IN_PROGRESS |
Another authentication or enrollment operation is processing. This can happen when your application logic calls more than once enroll() or authenticate() due to poor UI implementation on your side (eg. User taps twice the same button triggering the facial authentication process).Starting with fio.js 1.9 , it is possible now to restart the current user session without reloading the entire HTML page via simple call to the restartSession() method. |
Ongoing operation is still processing and the FACEIO Widget continue running. Your error handler routine should probably ignore this error code as the operation is still ongoing. |
fioErrCode.FACE_DUPLICATION |
Introduced in version 1.9 This error code is raised when the same user tries to enroll a second time on your application. That is, his facial features are already recorded due to previous enrollment, and can no longer enroll again due to the security setting: Prevent Same User from Enrolling Twice or More being activated. |
Ongoing enroll() operation is immediately aborted and control is transferred to the host application. |
fioErrCode.MINORS_NOT_ALLOWED |
Introduced in version 2.19 This error code is raised when a minor less than 18 years old try to onboard on your application following activation of the: Forbid Minors From Enrolling On This Application security setting. You may want to activate this feature if your application is offering sensitive services, and you need to comply with jurisdiction (eg: UK & Some US states) that forbid minors from accessing such services. PixLab software including Insight, the default facial recognition engine for FACEIO are ready to meet the PAS 1296:2018 code of practice for online age verification accredited by UK’s Age Check Certification Scheme (ACCS). |
Ongoing enroll() operation is immediately aborted and control is transferred to the host application. |
fioErrCode.TIMEOUT |
Ongoing operation timed out (eg, camera Access Permission, ToS Accept, Face not yet detected, Server Reply, etc.). | Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.NO_FACES_DETECTED |
No faces were detected during the enroll or authentication process. | Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.UNRECOGNIZED_FACE |
Unrecognized/unknown face on this application Facial Index. | Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.MANY_FACES |
Two or more faces were detected during the enroll or authentication process. | Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.PAD_ATTACK |
The face anti-spoofing system reported a Presentation attack (PAD) using a smartphone or printed picture for example during the authentication or enrollment process. For more information about Deep-Fakes & Face Anti-Spoofing prevention, and the technology behind it, please refer to our dedicated blog post here. |
Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.UNIQUE_PIN_REQUIRED |
Supplied PIN Code must be unique among other PIN's on this application. This warning code is raised only from the enroll() method, and only if you have enabled the Enforce PIN Code Uniqueness Security Option. |
Ongoing enroll() operation is still processing until the user being enrolled input a unique PIN code. |
fioErrCode.FACE_MISMATCH |
Calculated Facial Vectors of the user being enrolled do not matches. This error code is raised only from the enroll() method. |
Ongoing enroll() operation is immediately aborted and control is transferred to the host application. |
fioErrCode.WRONG_PIN_CODE |
Wrong PIN Code supplied by the user being authenticated. This error code is raised only from the authenticate() method. |
Ongoing authenticate() operation is immediately aborted after three trials and control is transferred to the host application. |
fioErrCode.NETWORK_IO |
Error while establishing network connection with the FACEIO processing node. | Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.PROCESSING_ERR |
Server side error. | Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.UNAUTHORIZED |
Your application is not allowed to perform the requested operation (eg. Invalid ID, Blocked, Paused, etc.). Refer to the FACEIO Console for additional information. | Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.UI_NOT_READY |
The FACEIO fio.js library could not be injected onto the client DOM. |
Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.TOO_MANY_REQUESTS |
Widget instantiation requests exceeded for freemium applications. Does not apply for premium applications as fio.js instantiation is unmetered. |
Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.EMPTY_ORIGIN |
Origin or Referer HTTP request header is empty or missing while instantiating fio.js .This error is raised only if you have enforced the Reject Empty Origin Security Option. |
Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.FORBIDDDEN_ORIGIN |
Domain origin is forbidden from instantiating fio.js .This error is raised only if you have created a white list of authorized domain names via the FACEIO Console. |
Ongoing operation is immediately aborted and control is transferred to the host application. |
fioErrCode.FORBIDDDEN_COUNTRY |
Country ISO-3166-1 Code is forbidden from instantiating fio.js .This error is raised only if you have created a white list of authorized countries via the FACEIO Console. |
Ongoing operation is immediately aborted and control is transferred to the host application. |
HTML Integration Boilerplate
The HTML boilerplate below should serve as a reference when implementing FACEIO on your website for the first time. The same code is available to consult via this Github Gist. Do not forget to replace the dummy field on the boilerplate with your real application Public ID when you instantiate fio.js
. Your application Public ID is located at the Application Manager on the FACEIO Console .
- Effective call to
enroll()
&authenticate()
are made respectively on line 31, and 60 of the gist above. The JavaScript code is self explanatory, and you should be able to understand the ongoing logic here at this stage. If you have any trouble, please refer to the documentation above or our getting started guide. - Feel free to modify the boilerplate code, and adjust it to your need especially with the
payload
parameter theenroll()
method takes which is used to associate arbitrary data to the newly enrolled user. This payload will be forwarded back to you upon future authentication of this particular user. Refer to theenroll()
. fio.js
require a running HTTP server to load. Otherwise, you won't be able to invoke the Widget due to missing HTTP security headers. Simply drop the boilerplate on your server HTML directory, and access it from your favorite browser. Refer to our Security Best Practices guide for additional information.
Community Contributed Tutorials
Learn how to use FACEIO for a variety of use cases. The following, high-content, community contributed guides & tutorials should help you implement fio.js
on your mobile (React-Native or Flutter) or web application using your favorite JavaScript framework whether it is React, Vue, Angular, Next, React or Vanilla JavaScript:
- Official NPM Package for FACEIO ↗
- FACEIO Community Forum - Find answers to the most common questions ↗
- Building an AI Vacation Planner Chatbot with Facial Authentication using FACEIO & Next.js ↗
- Build a Face Recognition Login System with FACEIO and Vanilla JavaScript ↗
- Streamlining Attendance Monitoring with FACEIO and Vanilla JavaScript ↗
- Building a Presence and Authentication System using face recognition with FACEIO and JavaScript ↗
- Building an Attendance System with Face Recognition Using Nextjs & FACEIO ↗
- Age Verification and Face Authentication with React & FACEIO ↗
- Building a Secure Event Booking App with FACEIO & Svelte ↗
- Facial Authentication & Recognition with Svelte and FACEIO ↗
- How to Authenticate a User with Face Recognition in React.js ↗
- Facial Authentication & Recognition with React.js, FACEIO & Tailwind CSS ↗
- Liveness Detection and Facial Authentication in JavaScript with FACEIO ↗
- Authenticate and verify users age in your Next.js application ↗
- Integration of Facial Authentication (Recognition) on an Employee Dashboard using FACEIO, Next.js & Typescript ↗
- Facial Authentication with FACEIO & HTMX ↗
- Implement a Facial Recognition Authentication System Using React.js & Tailwind ↗
- Build a Face Recognition & Authentication System for Your Website using Next.js & FACEIO ↗
- FACEIO - New Age Face Authentication ↗
- Authenticate Users with Face Recognition in React.js ↗
- Enhance user Experience with Face Recognition ↗